1. DOM scripting introduction
DOM
is the browser's internal representation of the document's HTML structure as a hierarchy of objects.- The important parts of a web browser as represented in JavaScript
Navigator
,Window
, andDocument
. - DOM nodes exist relative to each other in the DOM tree
root
,parent
,child
,sibling
, anddescendant
.- Nodes are also referred to by their position in the tree relative to other nodes:
Root node
: The top node in the tree, which in the case of HTML is always the HTML nodeDescendant node
: A node anywhere inside another node.Parent node
: A node which has another node inside it.Child node
: A node directly inside another node.Sibling nodes
: Nodes that sit on the same level under the same parent node in the DOM tree.
- Nodes are also referred to by their position in the tree relative to other nodes:
- For more go to Javascript DOM file in Document.
2. Asynchronous JavaScript
1. Making network requests with JavaScript
- A web page consists of an HTML page and (usually) various other files, such as stylesheets, scripts, and images.
- The basic model of page loading on the Web is that your
browser
makes one or moreHTTP requests
to theserver
for the files needed to display the page, and the server responds with the requested files. - If you visit another page, the browser requests the new files, and the server responds with them.
- The
trouble with the traditional model
here is that we'd have tofetch and load the entire page
, even when we only need to update one part of it. - So instead of the traditional model, many websites use
JavaScript APIs
to request data from the server and update the page content without a page load. - So when the user searches for a new product, the browser only requests the data which is needed to update the page.
- The main API here is the
Fetch API
. - This enables JavaScript running in a page to make an HTTP request to a server to retrieve
specific resources
. - When the server provides them, the JavaScript can use the data to update the page, typically by using
DOM manipulation APIs
. - The data requested is often
JSON
, which is a good format for transferring structured data, but can also beHTML
or justtext
. - In the early days, this general technique was known as
Asynchronous JavaScript and XML (AJAX)
.
2. API
API (Application Programming Interface) is a way for different software systems to communicate with each other.
Types of APIs
- 1. Browser APIs (JavaScript-Specific) - APIs built into the browser for interacting with web features.
DOM API (Document Object Model)
- Manipulates HTML elements.Web Storage API
- Stores data in the browser (localStorage, sessionStorage).Fetch API
- Retrieves data from a URL.Geolocation API
- Gets user's location.
- 2. Web APIs - Used to fetch data from the internet (e.g., weather, user info).
REST APIs
- The most common type, using HTTP requests like GET, POST, PUT, and DELETE.GraphQL APIs
- Allows you to request only the data you need.SOAP APIs
- Uses XML for messaging. More secure than REST but less popular now.
- 3. Operating System APIs (Outside JavaScript) - APIs that allow software to interact with the OS.
Windows API (WinAPI)
-Used for creating Windows applications. Used In: C++, C#.POSIX API (Unix/Linux)
- System calls for Unix-based OS operations. Used In: C, C++.macOS/iOS APIs (Cocoa, Swift)
- Used for Apple applications. Used In: Swift, Objective-C.
- 4. Hardware APIs (Used Outside JavaScript) - APIs that let software interact with hardware devices.
DirectX & OpenGL (Graphics APIs)
- Used for rendering graphics (gaming, 3D apps). Used In: C++, Python, Java.Vulkan API
- Low-overhead, high-performance graphics API. Used In: C++, Rust.Bluetooth API
- Used to communicate with Bluetooth devices. Used In: Python, Java, Swift.
- 5. Cloud & Third-Party APIs- APIs provided by third-party services for cloud computing, payments, and social media.
Google Cloud API
- Provides cloud services (storage, AI, etc.). Used In: JavaScript, Python, Java.Stripe API
- Handles online payments.Used In: JavaScript, Python, PHP.Twitter API
- Accesses tweets and user data. Used In: JavaScript, Python.
- 1. Browser APIs (JavaScript-Specific) - APIs built into the browser for interacting with web features.
How to implement a promise-based API
Introducing workers
- Workers give you the ability to run some tasks in a different thread, so you can start the task, then continue with other processing (such as handling user actions).
- There are three different sorts of workers:
dedicated workers
shared workers
service workers
Shared workers
can be shared by several different scripts running in different windows.Service workers
act like proxy servers, caching resources so that web applications can work when the user is offline.
3. Client-side web API
- Application Programming Interfaces (APIs) are programming features for manipulating different aspects of the browser and operating system the site is running on, or manipulating data from other websites or services.
Introduction to web APIs
Video and audio APIs
Drawing graphics
Client-side storage
Third party APIs
- For more go to Asynchronous JavaScript file in Document.
3. JavaScript Data Structure
- Dynamic and weak typing
- JavaScript is also a weakly typed language, which means it allows implicit type conversion when an operation involves mismatched types, instead of throwing type errors.
- Primitive values
- All types except Object define immutable values represented directly at the lowest level of the language.
- We refer to values of these types as primitive values. Null, Undefined, Boolean, Number, BitInt, String, Symbol.
- Objects
- Properties: There are two types of object properties:
data property
and theaccessor property
.- Data property
value
: The value retrieved by a get access of the property. Can be any JavaScript value.writable
: A boolean value indicating if the property can be changed with an assignment.enumerable
: A boolean value indicating if the property can be enumerated by a for…in loop.configurable
: A boolean value indicating if the property can be deleted, can be changed to an accessor property, and can have its attributes changed.
- Accessor property
get
: A function called with an empty argument list to retrieve the property value whenever a get access to the value is performed. See also getters. May be undefined.set
: A function called with an argument that contains the assigned value. Executed whenever a specified property is attempted to be changed. See also setters. May be undefined.enumerable
: A boolean value indicating if the property can be enumerated by a for…in loop. See also Enumerability and ownership of properties for how enumerability interacts with other functions and syntaxes.configurable
: A boolean value indicating if the property can be deleted, can be changed to a data property, and can have its attributes changed.
- Data property
- Dates
- JavaScript provides two sets of APIs for representing dates: the
legacy Date
object and themodern Temporal
object.
- JavaScript provides two sets of APIs for representing dates: the
- Indexed collections: Arrays and typed Arrays
Arrays
are regular objects for which there is a particular relationship between integer-keyed properties and the length property.Typed Arrays
present an array-like view of an underlying binary data buffer, and offer many methods that have similar semantics to the array counterparts.
- Keyed collections: Maps, Sets, WeakMaps, WeakSets
- These data structures take object references as keys.
- Set and WeakSet represent a collection of unique values.
- while Map and WeakMap represent a collection of key-value associations.
- Structured data: JSON
- JSON (JavaScript Object Notation) is a lightweight data-interchange format.
- derived from JavaScript, but used by many programming languages.
- JSON builds universal data structures that can be transferred between different environments and even across languages.
- For more go to this MDN Page.
- Properties: There are two types of object properties:
- Type Coercion
Primitive coercion
Numeric coercion
string coercion
boolean coercion
object coercion
4. Equality comparisons and sameness
=== strict equality (triple equals)
- Double equals (==) will perform a type conversion when comparing two things,
- and will handle NaN, -0, and +0 specially to conform to IEEE 754 (so NaN != NaN, and -0 == +0);
== loose equality (double equals)
- Triple equals (===) will do the same comparison as double equals (including the special handling for NaN, -0, and +0)
- but without type conversion; if the types differ, false is returned.
Object.is()
Object.is()
does no type conversion and no special handling for NaN, -0, and +0.- (giving it the same behavior as === except on those special numeric values).
Comparing equality methods
x y == === Object.is() SameValueZero undefined undefined ✅ true ✅ true ✅ true ✅ true null null ✅ true ✅ true ✅ true ✅ true true true ✅ true ✅ true ✅ true ✅ true false false ✅ true ✅ true ✅ true ✅ true 'foo' 'foo' ✅ true ✅ true ✅ true ✅ true 0 0 ✅ true ✅ true ✅ true ✅ true +0 -0 ✅ true ✅ true ❌ false ✅ true +0 0 ✅ true ✅ true ✅ true ✅ true -0 0 ✅ true ✅ true ❌ false ✅ true 0n -0n ✅ true ✅ true ✅ true ✅ true 0 false ✅ true ❌ false ❌ false ❌ false “” false ✅ true ❌ false ❌ false ❌ false “” 0 ✅ true ❌ false ❌ false ❌ false ‘0’ 0 ✅ true ❌ false ❌ false ❌ false ‘17’ 17 ✅ true ❌ false ❌ false ❌ false [1, 2] ‘1,2’ ✅ true ❌ false ❌ false ❌ false new String(‘foo’) ‘foo’ ✅ true ❌ false ❌ false ❌ false null undefined ✅ true ❌ false ❌ false ❌ false null false ❌ false ❌ false ❌ false ❌ false undefined false ❌ false ❌ false ❌ false ❌ false {foo: ‘bar’ } {foo: ‘bar’ } ❌ false ❌ false ❌ false ❌ false new String(‘foo’) new String(‘foo’) ❌ false ❌ false ❌ false ❌ false 0 null ❌ false ❌ false ❌ false ❌ false 0 NaN ❌ false ❌ false ❌ false ❌ false ‘foo’ NaN ❌ false ❌ false ❌ false ❌ false NaN NaN ❌ false ❌ false ✅ true ✅ true
5. Enumerability and ownership of properties
Every property in JavaScript objects can be classified by three factors:
Enumerable
ornon-enumerable
;Enumerable
properties are those properties whoseinternal enumerable flag
is set totrue
.Enumerable
properties show up in loops likefor...in and Object.keys()
.- Property that do not show up in
for...in or Object.keys()
arenon-enumerable
.
// Enumerable const obj = {name: "Alice", age: 25 }; console.log(Object.keys(obj)); // ["name", "age"] for (let key in obj) { console.log(key); // name, age } // Non-Enumerable const person = {name: "Alice" }; Object.defineProperty(person, "age", { value: 25, enumerable: false, // Hidden from loops }); console.log(Object.keys(person)); // ["name"] for (let key in person) { console.log(key); // "name" (age is hidden) } console.log(person.age); // 25 (still accessible directly)
String
orsymbol
;Own property
orinherited property
from the prototype chainAll
properties, enumerable or not, string or symbol, own or inherited,can be accessed
withdot notation
orbracket notation
.
Property Type \ Methods propertyIsEnumerable() hasOwnProperty() Object.hasOwn() in Enumerable, Own ✅ true
✅ true
✅ true
✅ true
Enumerable, Inherited ❌ false
❌ false
❌ false
✅ true
Non-Enumerable, Own ❌ false
✅ true
✅ true
✅ true
Non-Enumerable, Inherited ❌ false
❌ false
❌ false
✅ true
6. Closure
Lexical scope
is like a backpack a function carries with it - it can access variables from where it was written.Closures
are like a saved memory - even if the original scope is gone, the function still remembers and can use it's variables.Lexical scoping
- The word lexical refers to the fact that lexical scoping uses the location where a variable is declared within the source code to determine where that variable is available.
function init() { let name = "Mozilla"; // name is a local variable created by init function displayName() { // displayName() is the inner function, that forms a closure console.log(name); // use variable declared in the parent function } displayName(); } init(); // Mozilla
Closure
- A closure gives a function access to its outer scope even after the outer function is finished executing.
- In JavaScript, closures are created every time a function is created, at function creation time.
function makeFunc() { const name = "Mozilla"; function displayName() { console.log(name); } return displayName; // here it will return the lexical scope of displayName // (whole makeFunc function's scope { }) not only the executional context. } const myFunc = makeFunc(); myFunc();
Practical Implementation
document.getElementById("orange").onclick = function(){ document.body.style.backgroundColor = "orange" } document.getElementById("green").onclick = function(){ document.body.style.backgroundColor = "orange" } function clickHandler(color){ return function () { document.body.style.backgroundColor = `${color}` } // the inner function is being returned but because of, // closure the function can remember and use the "color" argument. } document.getElementById("blue").onclick = clickHandler("blue")
7. Concurrency model
In programming, a concurrency model defines how a language or framework handles multiple tasks executing at the same time.
It ensures safe and efficient execution of concurrent operations.
Thread-Based Model
: Uses multiple threads sharing memory, requiring synchronization (e.g., Java Threads, C++ std::thread).-
Message Passing Model
: Tasks communicate via messages instead of shared memory(e.g., Erlang’s Actor Model, Go’s channels). -
Event-Driven Model
: Uses an event loop and non - blocking I / O(e.g., Node.js, Python’s asyncio). -
Coroutine-Based Model
: Lightweight concurrency using cooperative multitasking (e.g., Python’s async / await, Kotlin coroutines). -
Dataflow Model
: Execution depends on data dependencies(e.g., TensorFlow, Apache Spark).
8. Hoisting and Execution Context
Execution Context
- An execution context is an environment in which JavaScript source code is executed.
- It contains all the information required to execute the code, such as variables, functions, and the value of this.
1.Global Execution Context
Global Execution Context
- The default context where any JavaScript source code starts executing.
- It creates the global object (in browsers, it's window).
- All variables and functions declared in the global scope are added to the global object.
Only one global execution context
exists in any JavaScript program.
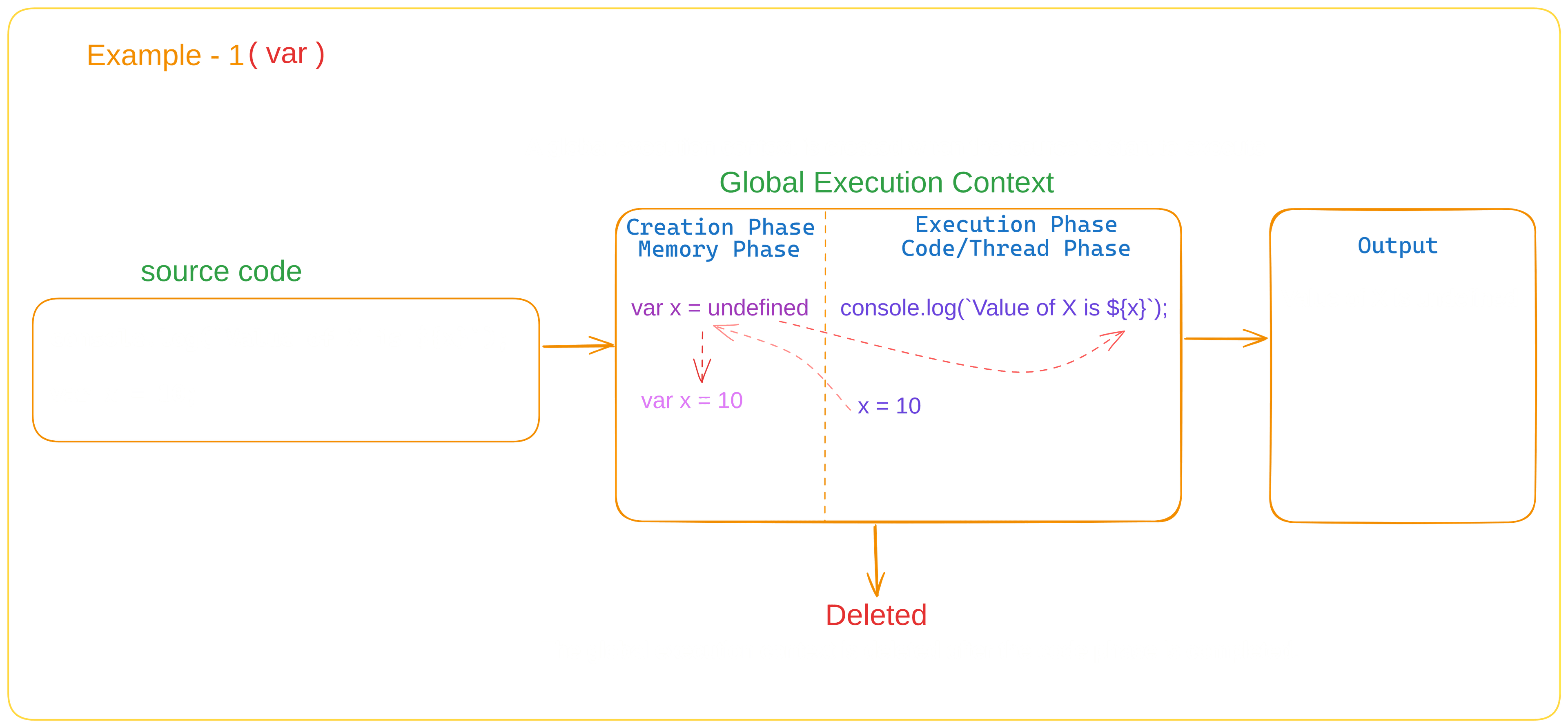
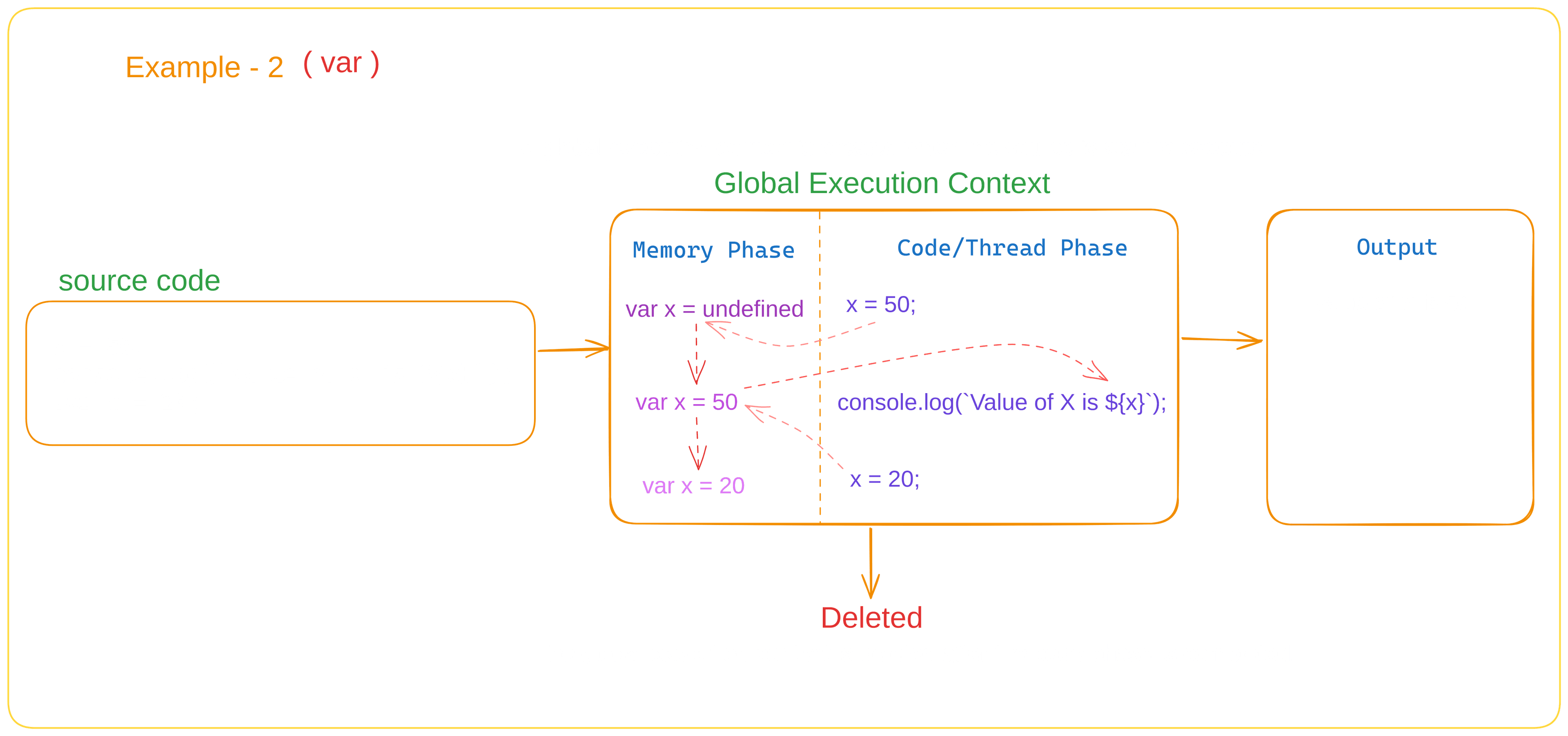
Hoisting
- Hoisting is a JavaScript mechanism where variable and function declarations are moved (“hoisted”) to the top of their scope before code execution.
- However, only the declarations are hoisted, not the initializations.
Function Hoisting
: Function declarations are fully hoisted, meaning they can be called before their actual definition in the code.Variable Hoisting
: Variables declared withvar
are hoisted but initialized asundefined
.let & const Hoisting
: On the other hand,let
andconst
are also hoisted withundefined
,- but
let
andconst
remain in theTemporal Dead Zone (TDZ)
until their declaration is encountered.
Temporal Dead Zone (TDZ)
- The
Temporal Dead Zone (TDZ)
is the period between thestart of execution
and theactual declaration
of a let or const variable. - During this time, accessing the variable results in a
ReferenceError
. - Since
const
variables must be initialized at the time of declaration, they also have a TDZ. TDZ prevents
the use of variables before they are properly initialized, making the code more predictable and reducing potential bugs.
- The
Hoisting
Temporal Dead Zone (TDZ)
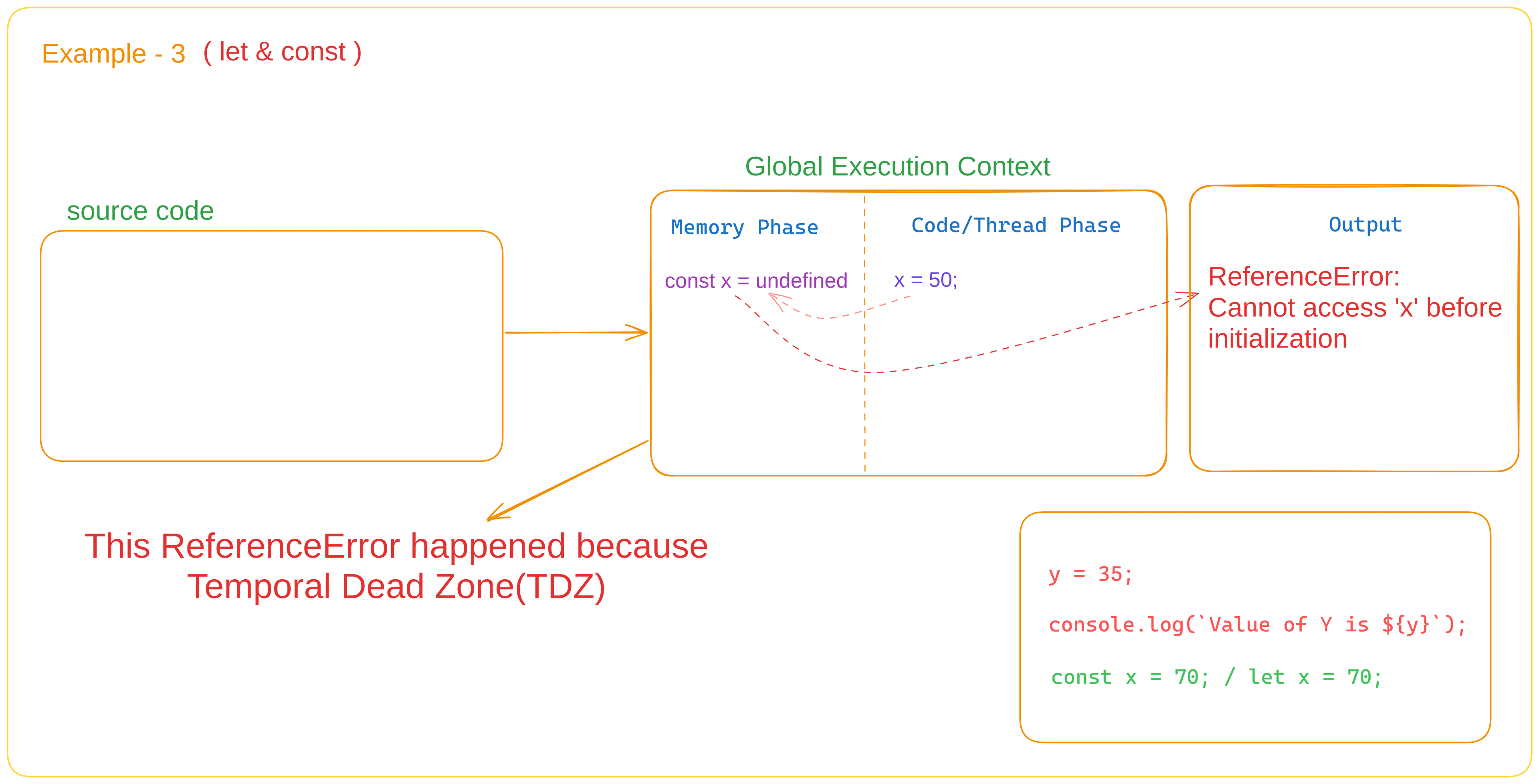
2.Function Execution Context
Function Execution Context
- Every time a function is invoked, a new execution context is created for that function.
- A new variable environment is created for each function call to store local variables and parameters.
- The value of this is set based on how the function is called.
Eval Execution Context
- A special execution context created when eval() is executed.
- when eval function is called.
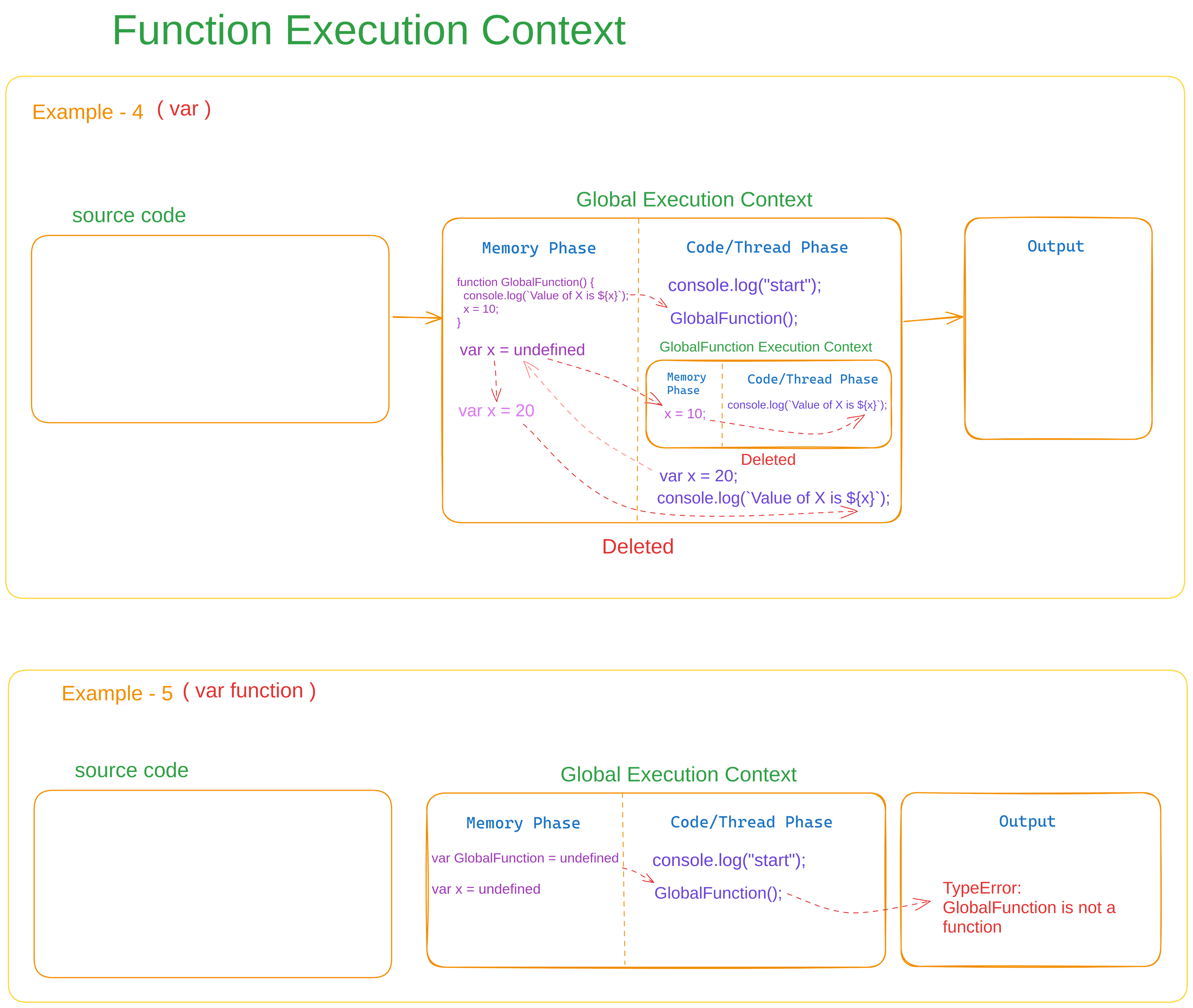
Execution Context Phase Actions Global Execution Context (GEC) Memory Creation Phase Variables set to undefined
Functions stored in memory Code Execution Phase Executes top-level code Calls Function A Function Execution Context (FEC) Memory Creation Phase Function variables set to undefined
Inner function stored in memory Code Execution Phase Executes function code
9. Call Stack and Event loop
- The event loop is responsible for handling asynchronous operations and ensuring smooth execution.
- Checking Queues: The event loop continuously checks these queues and moves ready tasks to the call stack for execution.
- Execution & Repeat: The cycle repeats indefinitely.
- The call stack is a LIFO (Last In, First Out) data structure where JavaScript keeps track of function calls.
- When a function is invoked, it's pushed onto the stack.
- When a function completes execution, it's popped off the stack.
- JavaScript is single-threaded, meaning only one function executes at a time.
- When an async task (like setTimeout, setInterval, or I/O operations) completes, its callback moves to the Callback Queue.
- The event loop will push tasks from this queue into the Call Stack only when the stack is empty.
- Microtasks include Promises, MutationObserver, and queueMicrotask().
- The Microtask Queue has higher priority than the Callback Queue.
- After each task (function execution) completes, the event loop first clears the Microtask Queue before moving to the Callback Queue.
- If the Microtask Queue keeps filling up
(e.g., continuous Promise.then() calls), the Callback Queue (e.g., setTimeout)
may never execute. - This is called
“starvation”
, as lower-priority tasks are never processed.
- If the Microtask Queue keeps filling up
- JavaScript in browsers doesn't handle everything alone—it delegates tasks
- like
setTimeout, DOM events,
andAJAX requests to Web APIs
. - These APIs handle operations asynchronously without blocking the main thread.
1. event loop
2. Call Stack (Execution Stack)
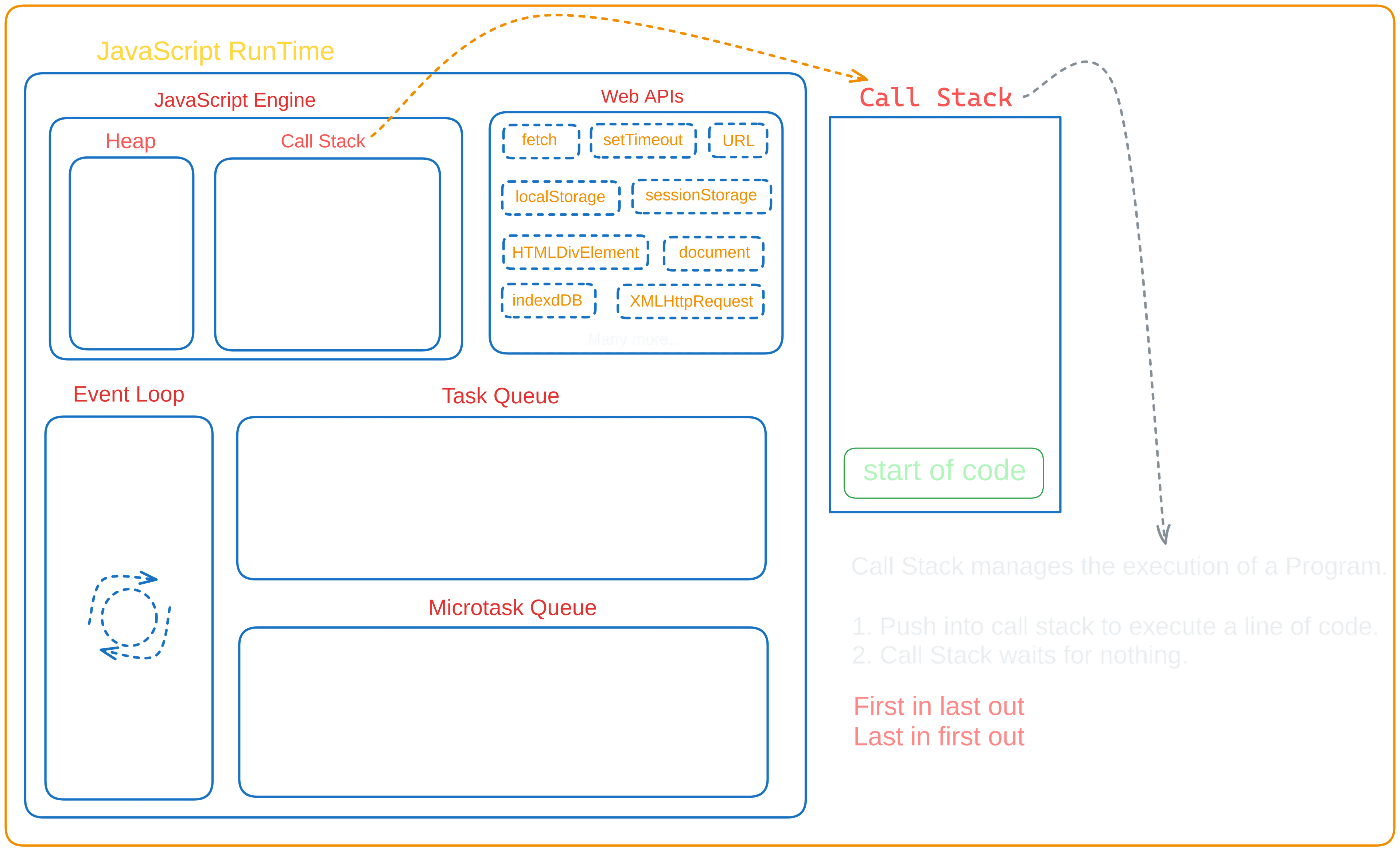
Callback Queue (Task Queue)
Microtask Queue (Priority Queue)
Callback and Promise based APIs Example:
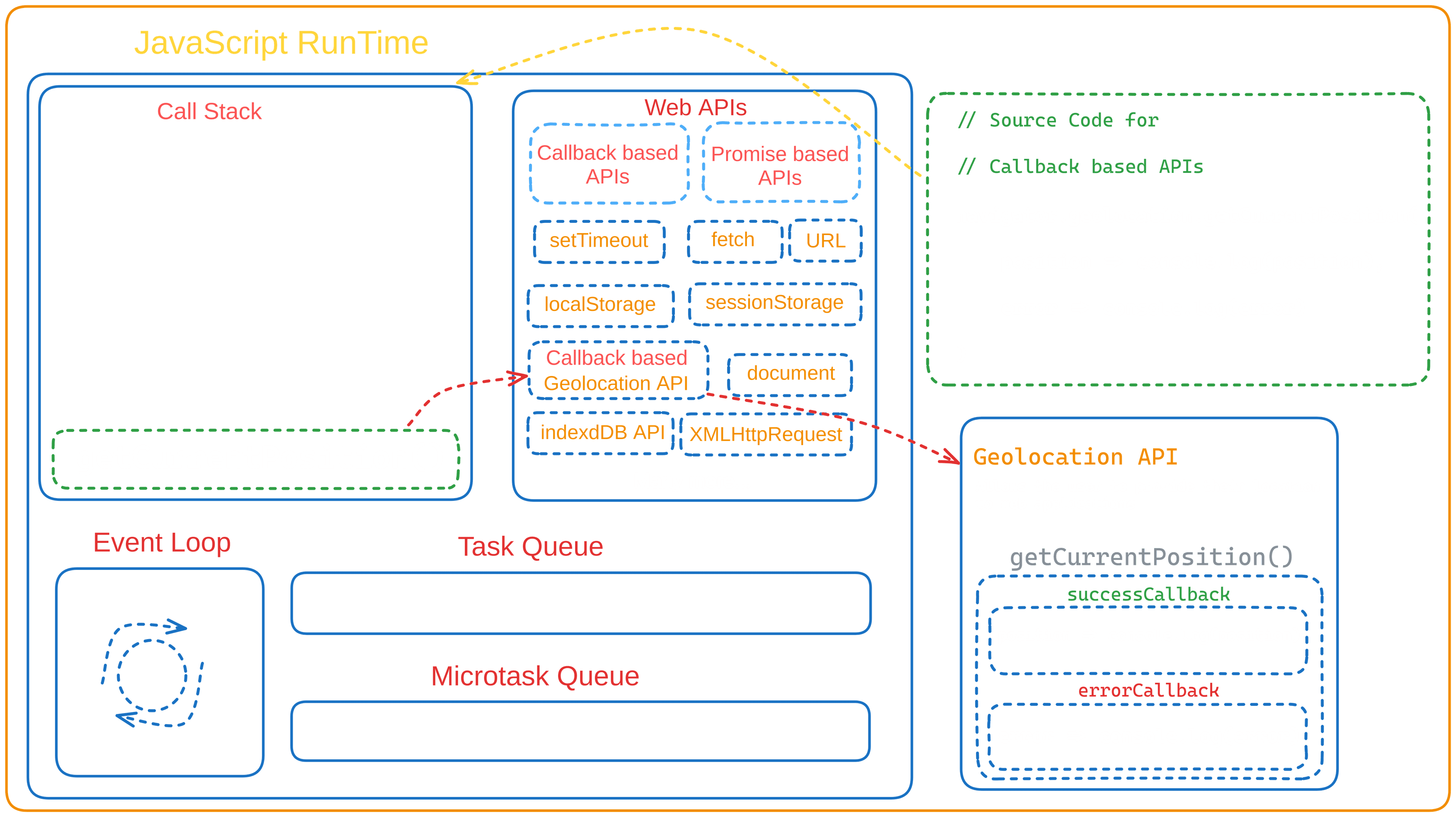
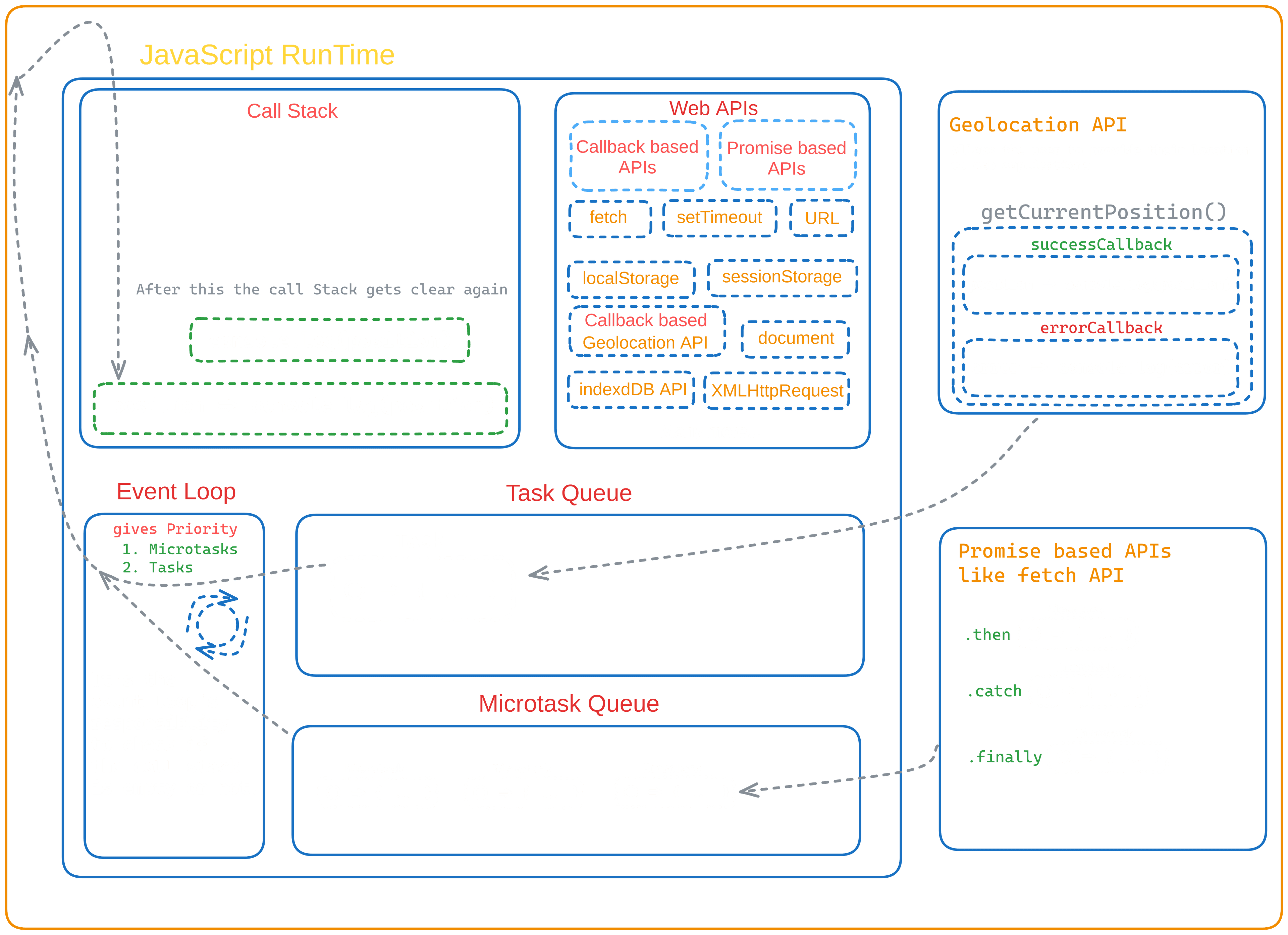
Starvation (Priority Inversion)
Web APIs
10. Memory management
1. Memory Lifecycle in JavaScript
Memory management follows three key stages:
- 1.
Allocation
: Memory is allocated when a variable, object, or function is created. - 2.
Usage
: The allocated memory is used during program execution (e.g., reading/writing data). - 3.
Deallocation(Garbage Collection)
: Unused memory is automatically freed by JavaScript.
- 1.
2. Type of Memory
JavaScript handles data types in memory using two primary storage locations:
- 1.
Stack
(for primitive types) - 2.
Heap
(for reference types)
- 1.
Stack Memory (For Primitive Types)
- Stack memory is used for storing primitive values and function call execution.
- These datatypes are immutable(cannot be modified) and stored directly in memory.
- It operates on LIFO(Last In, First Out) order.
- Data stored in the stack is fast and automatically managed(garbage collected).
let x = 10; let y = x; // Copying value, NOT reference console.log(x); // 10 console.log(y); // 10 y = 20; // Changing y does NOT affect x console.log(x); // 10 console.log(y); // 20 x = 12; // Changing x does NOT affect y console.log(x); // 12 console.log(y); // 20
Heap Memory (For Reference Types)
- Heap memory is used for storing objects, arrays, and functions.
- Objects are mutable(modifications affect all references to the object).
- These hold references to memory locations instead of storing values directly.
- Reference types store a memory address (reference) in the stack, while the actual value is stored in the heap.
- Data stored in the heap is slower and manually managed(garbage collected).
let obj1 = {name: "Alice" }; let obj2 = obj1; // Both variables point to the same memory location obj2.name = "Bob"; // Changing obj2 affects obj1 console.log(obj1.name); // Output: "Bob" console.log(obj2.name); // Output: "Bob" obj1.name = "Sam"; // And changing obj1 affects obj2 console.log(obj1.name); // Output: "Sam" console.log(obj2.name); // Output: "Sam"
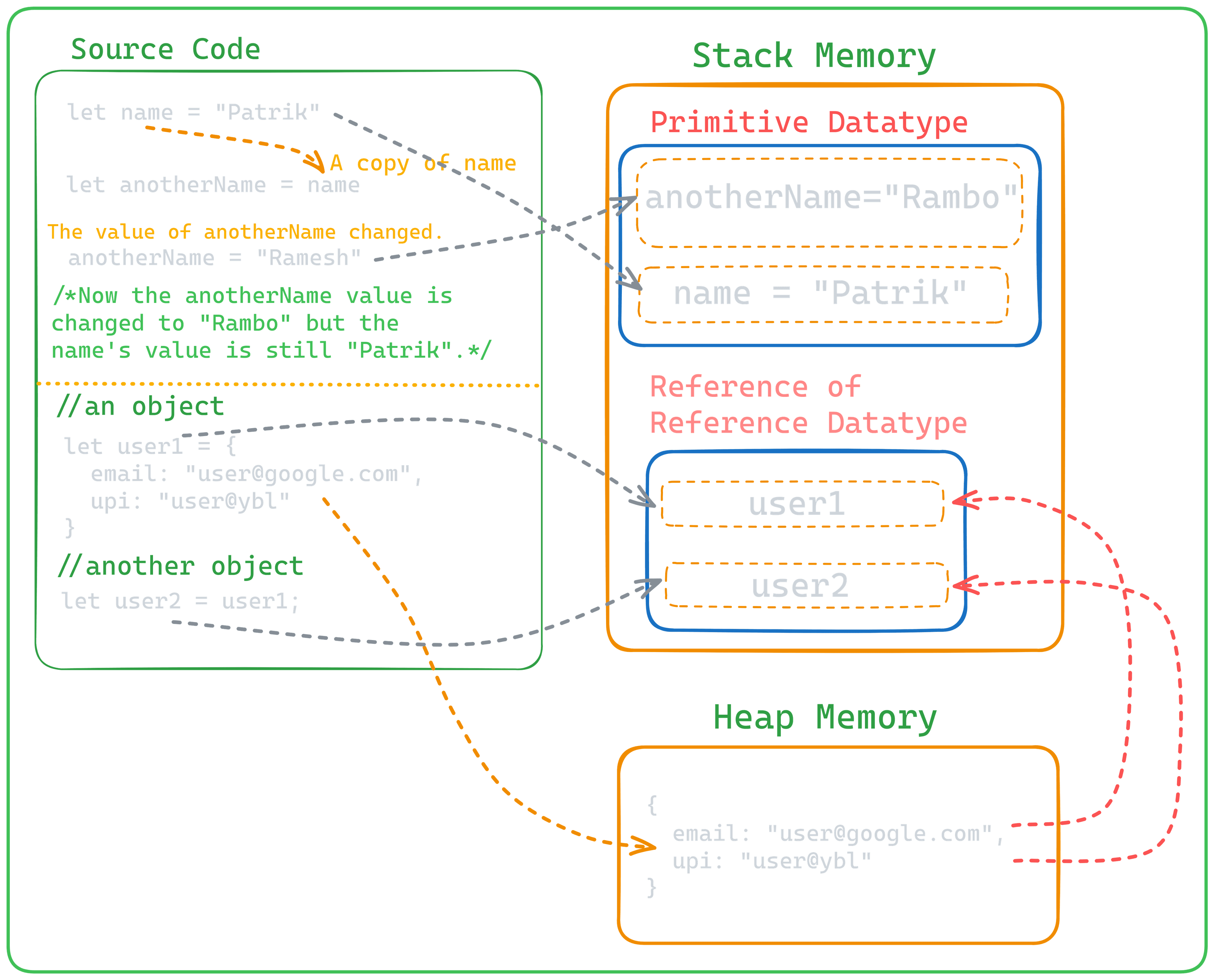
3. Garbage Collection in JavaScript
Since JavaScript doesn't have explicit memory management, it uses
Garbage Collection (GC)
to automatically free unused memory.How Garbage Collection Works: The most common algorithm is
Mark - and - Sweep
:Mark Phase
:The GC finds all objects that are reachable (still referenced).Sweep Phase
: Unreachable objects (not referenced) are removed from memory.A value is
reachable
if:- - It is in the global scope.
- - It is referenced by another reachable object.
- - It is on the call stack.
4. Common Memory Issues
1.
Memory Leak: Unused Global Variables
- Global variables persist in memory until the page reloads.
function leak() { globalVar = "I am a leak"; // No 'let', 'const', or 'var' (becomes a global variable) } leak();
Solution
: Always use let, const, or var to prevent accidental globals.
2.
Memory Leak: Unused Event Listeners
- If event listeners aren't removed, they hold references to objects, preventing garbage collection.
document.getElementById("btn").addEventListener("click", () => { console.log("Clicked"); }); // This persists forever unless removed
Solution
: Remove event listeners when they are no longer needed.
const button = document.getElementById("btn"); function handleClick() { console.log("Clicked"); button.removeEventListener("click", handleClick); } button.addEventListener("click", handleClick);
3.
Memory Leak: Closures Holding References
- Closures keep variables in memory even after the function execution is done.
function outer() { let largeArray = new Array(1000000).fill("data"); return function inner() { console.log(largeArray.length); // Still holds reference }; } const closure = outer(); // largeArray is never garbage-collected
Solution
: Set large objects to null when they are no longer needed.
function outer() { let largeArray = new Array(1000000).fill("data"); return function inner() { console.log(largeArray.length); largeArray = null; // Allow garbage collection }; } const closure = outer();
4.
Memory Leak: DOM Elements Not Removed
- If you store references to DOM elements and don't remove them when they're detached, memory leaks occur.
let div = document.createElement("div"); document.body.appendChild(div); div.remove(); // Removes from DOM but not from memory
Solution
: Set references to null after removing elements.
div.remove(); div = null;
5.
WeakMap and WeakSet
for Efficient Memory Usage- WeakMap and WeakSet store objects weakly, meaning they do not prevent garbage collection.
let wm = new WeakMap(); let obj = {name: "Alice" }; wm.set(obj, "some data"); obj = null; // Now 'obj' is eligible for garbage collection
11. this Keyword
this is a special keyword that refers to the execution context of a function.
Its value depends on how and where the function is called.
Global Execution Context this
In the global execution context (outside of any function), this refers to:
- window in browsers.
- global or globalThis in Node.js.
console.log(this); // In browser: Window {...}
Object Method this
- When this is used inside an object method, it refers to the object itself.
const obj = { name: "Alice", greet() { console.log(this.name); } }; obj.greet(); // "Alice"
Function this
- In regular functions (not inside objects), this refers to the global object (window in browsers, global in Node.js).
function showThis() { console.log(this); } showThis(); // window (in browser), global (in Node.js)
- In “use strict”, this in a function becomes undefined.
"use strict"; function showThis() { console.log(this); } showThis(); // undefined
Arrow Function this
- Arrow functions do not have their own this; they inherit it from their surrounding scope.
const obj = { name: "Bob", greet: function () { const arrowFn = () => console.log(this.name); arrowFn(); } }; obj.greet(); // "Bob" (inherits this from greet)
this in a Constructor Function
- When used inside a constructor function, this refers to the new instance.
function Person(name) { this.name = name; } const user = new Person("Charlie"); console.log(user.name); // "Charlie"
this in Class Methods
- In class methods, this refers to the instance of the class.
class User { constructor(name) { this.name = name; } greet() { console.log(this.name); } } const user = new User("David"); user.greet(); // "David"
this inside an Event Listener
- In a DOM event listener, this refers to the element that triggered the event.
document.getElementById("btn").addEventListener("click", function () { console.log(this); // The button element });
- ( Arrow functions won't work the same way here because they don't have their own this. )
this in setTimeout and setInterval
- In setTimeout and setInterval, this usually refers to the global object.
const obj = { name: "John", sayName() { setTimeout(function () { console.log(this.name); // undefined (in strict mode) or window.name }, 1000); } }; obj.sayName(); // Fix using arrow function const obj2 = { name: "John", sayName() { setTimeout(() =& gt; { console.log(this.name); // "John" }, 1000); } }; obj2.sayName();
Explicit Binding (call, apply, bind)
- You can manually set this using:
- call()call(obj, arg1, arg2, ...) → Calls the function immediately with arguments.
- apply()apply(obj, [args]) → Same as call, but passes arguments as an array.
- bind()bind(obj, arg1, arg2, ...) → Returns a new function, which you call later.
const obj = {name: "Emma" }; function greet() { console.log(this.name); } greet.call(obj); // "Emma" greet.apply(obj); // "Emma" const boundFn = greet.bind(obj); boundFn(); // "Emma" const obj = { num: 10 };
function add(x) { return this.num + x; } console.log(add.call(obj, 5)); // call: 15 console.log(add.apply(obj, [5])); // apply: 15 console.log(add.bind(obj, 5)()); // bind: 15